Interstage Application Server アプリケーション作成ガイド (CORBAサービス編)
|
目次
索引

|
6.16.6 AdapterActivator使用例 (リクエスト受信時)
(1)IDL定義
module ODsample{
interface intf1{
long add( in long a, in long b );
};
interface intf2{
long sub( in long a, in long b );
};
};
(2)ネーミングサービスの登録
OD_or_adm -c IDL:ODsample/intf1:1.0 -a Imple_POAsample10 -n ODsample::POAsample10-1
OD_or_adm -c IDL:ODsample/intf2:1.0 -a Imple_POAsample10 -n ODsample::POAsample10-2
(3)アプリケーション構成概要図
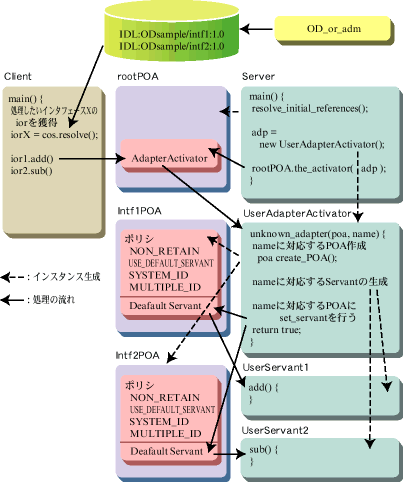
(4)クライアントアプリケーション
import java.io.*;
import org.omg.CORBA.*;
import org.omg.CosNaming.*;
import ODsample.*;
public class Client {
public static void main( String args[] ) {
int in1 = 0; // inパラメタ用変数
int in2 = 0; // inパラメタ用変数
String line = null;
try {
// ORBの生成と初期化
ORB Orb = ORB.init( args, null );
// ネーミングサービスのオブジェクトリファレンスの取得
org.omg.CORBA.Object _tmpObj =
Orb.resolve_initial_references( "NameService" );
NamingContextExt Cos = NamingContextExtHelper.narrow( _tmpObj );
// ネーミングサービスのresolveメソッドを発行し、
// サーバアプリケーションのオブジェクトリファレンスの獲得
String NCid =
new String( "ODsample::POAsample10-1" ); // オブジェクト名
String NCkind = new String( "" ); // オブジェクトタイプ
NameComponent nc = new NameComponent( NCid, NCkind );
NameComponent NCo[] = { nc };
org.omg.CORBA.Object Obj = Cos.resolve( NCo );
// サーバアプリケーションのオブジェクトリファレンス獲得
intf1 _intf1 = intf1Helper.narrow( Obj );
try {
System.out.print( "in1 => " );
line =
new BufferedReader( new InputStreamReader( System.in ) ).readLine();
in1 = Integer.parseInt( line );
System.out.print( "in2 => " );
line =
new BufferedReader( new InputStreamReader( System.in ) ).readLine();
in2 = Integer.parseInt( line );
}
catch ( java.lang.NumberFormatException e ) {
System.exit( 255 );
}
// サーバアプリケーションのメソッド呼出し
int result = _intf1.add( in1, in2 );
// メソッドの結果表示
System.out.println( in1 + " + " + in2 + " = "+ result );
// ネーミングサービスのresolveメソッドを発行し、
// サーバアプリケーションのオブジェクトリファレンスの獲得
String NCid2 =
new String( "ODsample::POAsample10-2" ); // オブジェクト名
String NCkind2 = new String( "" ); // オブジェクトタイプ
NameComponent nc2 = new NameComponent( NCid2, NCkind2 );
NameComponent NCo2[] = { nc2 };
org.omg.CORBA.Object Obj2 = Cos.resolve( NCo2 );
// サーバアプリケーションのオブジェクトリファレンス獲得
intf2 _intf2 = intf2Helper.narrow( Obj2 );
try {
System.out.print( "in1 => " );
line =
new BufferedReader( new InputStreamReader( System.in ) ).readLine();
in1 = Integer.parseInt( line );
System.out.print( "in2 => " );
line =
new BufferedReader( new InputStreamReader( System.in ) ).readLine();
in2 = Integer.parseInt( line );
}
catch ( java.lang.NumberFormatException e ) {
System.exit( 255 );
}
// サーバアプリケーションのメソッド呼出し
result = _intf2.sub( in1, in2 );
// メソッドの結果表示
System.out.println( in1 + " - " + in2 + " = "+ result );
}
catch( org.omg.CORBA.SystemException se ) {
System.out.println( "ERROR : " + se.getClass().getName()
+ " : minor = 0x" + java.lang.Integer.toHexString(se.minor) );
System.exit( 255 );
}
catch( org.omg.CORBA.UserException ue ) {
System.out.println( "ERROR : " + ue.getClass().getName() );
System.exit( 255 );
}
catch ( Exception e ) {
System.err.println( "ERROR : " + e );
System.exit( 255 );
}
}
}
(5)サーバアプリケーション
import org.omg.CORBA.*;
import org.omg.PortableServer.*;
import ODsample.*;
// ユーザアプリケーション:メイン処理クラス
public class Server {
public static void main(String args[]) {
try {
// ORBの生成と初期化
ORB Orb = ORB.init( args, null );
// RootPOAのオブジェクトリファレンスの取得
org.omg.CORBA.Object _tmpObj =
Orb.resolve_initial_references( "RootPOA" );
// RootPOAのPOAオブジェクト獲得
POA rootPOA = POAHelper.narrow( _tmpObj );
// AdapterActivatorの生成
AdapterActivator adp = new UserAdapterActivator();
// インプリ用POAのAdapterActivatorに設定
rootPOA.the_activator( adp );
// POAマネージャの獲得
POAManager poamanager = rootPOA.the_POAManager();
// POAマネージャのactivate
poamanager.activate();
Orb.run();
}
catch( org.omg.CORBA.SystemException se ) {
System.out.println( "ERROR : " + se.getClass().getName()
+ " : minor = 0x" + java.lang.Integer.toHexString(se.minor) );
System.exit( 255 );
}
catch( org.omg.CORBA.UserException ue ) {
System.out.println( "ERROR : " + ue.getClass().getName() );
System.exit( 255 );
}
catch ( Exception e ) {
System.err.println( "ERROR : " + e );
System.exit( 255 );
}
}
}
// アダプタアクティベータ:POA生成クラス(AdapterActivatorを継承)
// JDK/JRE1.3以前を使用する場合
// class UserAdapterActivator extends AdapterActivator {
// JDK/JRE1.4を使用する場合
class UserAdapterActivator extends LocalObject implements AdapterActivator {
public boolean unknown_adapter( POA parent, String name ) {
POA inf_POA; // インタフェース用POA
// ポリシリスト作成
org.omg.CORBA.Policy policies[] = new org.omg.CORBA.Policy[4];
policies[0] = parent.create_servant_retention_policy(
ServantRetentionPolicyValue.NON_RETAIN );
policies[1] = parent.create_request_processing_policy(
RequestProcessingPolicyValue.USE_DEFAULT_SERVANT );
policies[2] = parent.create_id_assignment_policy(
IdAssignmentPolicyValue.SYSTEM_ID );
policies[3] = parent.create_id_uniqueness_policy(
IdUniquenessPolicyValue.MULTIPLE_ID );
try {
if( name.equals( "IDL:ODsample/intf1:1.0" ) ) {
// intf1用のPOA作成
inf_POA = parent.create_POA( name, null, policies );
// Servantの生成
Servant svt = new UserServant1();
// Default Servantに設定
inf_POA.set_servant( svt );
return( true );
}
else if( name.equals( "IDL:ODsample/intf2:1.0" ) ) {
// intf2用のPOA作成
inf_POA = parent.create_POA( name, null, policies );
// Servantの生成
Servant svt = new UserServant2();
// Default Servantに設定
inf_POA.set_servant( svt );
return( true );
}
}
catch( Exception e ) {
System.err.println( "unknown_adapter error: " + e );
return( false );
}
return( true );
}
}
// Servant:メソッド実装クラス(スケルトンを継承)
class UserServant1 extends intf1POA {
public int add( int a, int b ) {
return( a + b );
}
}
class UserServant2 extends intf2POA {
public int sub( int a, int b ) {
return( a - b );
}
}
(6)例外情報の獲得
サーバアプリケーションで例外を獲得する方法は、クライアントアプリケーションの例外処理と同様です。詳細については、“クライアントアプリケーションの例外処理”を参照してください。
All Rights Reserved, Copyright(C) 富士通株式会社 2005